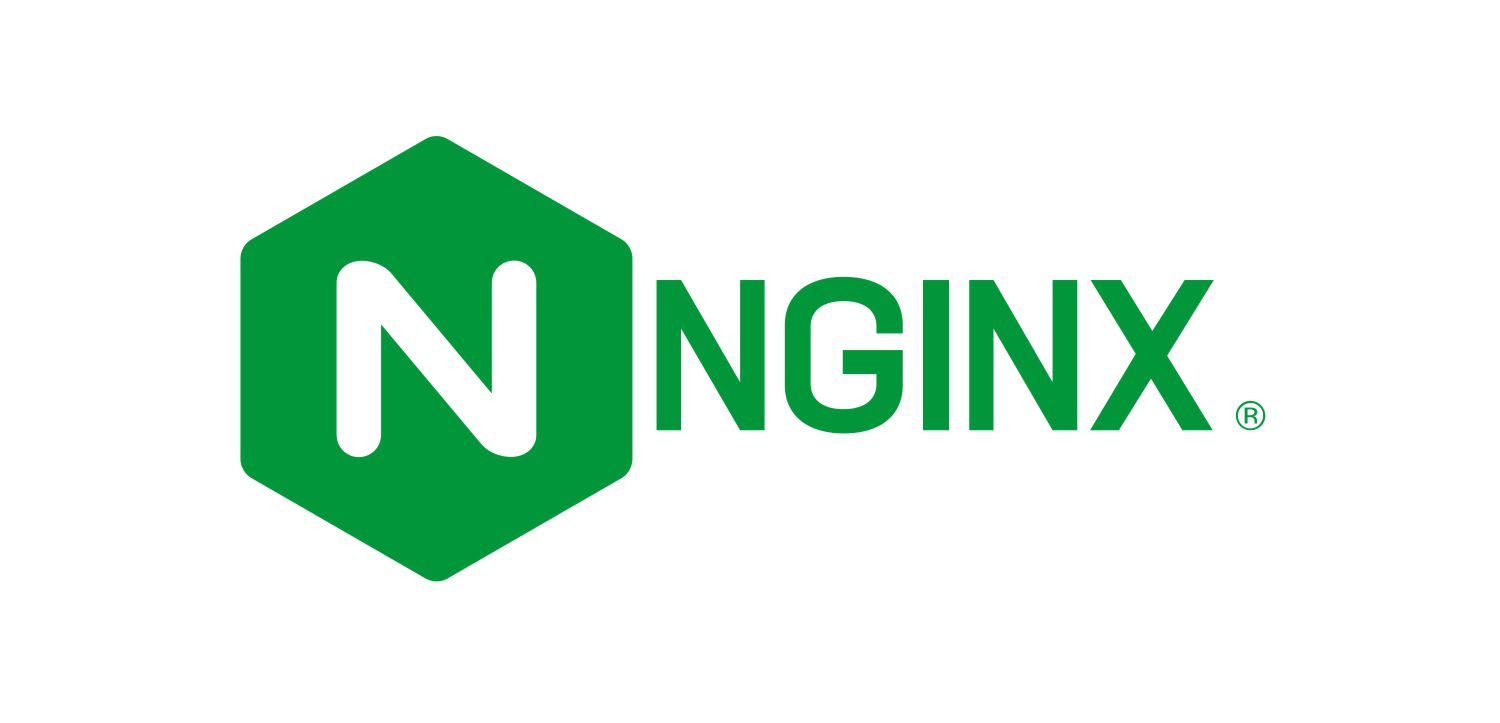
Nginx 常用命令
|
|
配置
端口
|
|
域名
|
|
静态资源
|
|
重定向
301 Permanent
|
|
302 Temporary
|
|
反向代理
正向代理和反向代理
系统内部要访问外部网络时,统一通过一个代理服务器把请求转发出去,在外部网络看来就是代理服务器发起的访问,此时代理服务器实现的是正向代理;
当外部请求进入系统时,代理服务器把该请求转发到系统中的某台服务器上,对外部请求来说,与之交互的只有代理服务器,此时代理服务器实现的是反向代理。
简单来说,正向代理是代理服务器代替系统内部来访问外部网络的过程,反向代理是外部请求访问系统时通过代理服务器转发到内部服务器的过程。
|
|
WebSockets
|
|
HTTPS
|
|
HTTP 重定向到 HTTPS
|
|
负载均衡
|
|
二级域名配置
添加解析
- 进入域名解析后台
- 添加记录
- 主机名:即二级域名的名字
- 解析记录:A
- 记录值:IP 地址,跟主域名一样配置
修改配置文件
|
|
重新加载配置文件即可访问二级域名
目录
相关文章
Linux
bash 目录操作 文件操作 进程管理 管道符 竖线 | ,在 linux 中是作为管道符的,将 | 前面命令的输出作为 | 后面的输入 1 grep
2018-1-12
Python
常规 Python 对大小写敏感 Python 的索引从 0 开始 Python 使用空白符(制表符或空格)来缩进代码,而不是使用花括号 帮助 获取主
2018-10-6
Go
安装 前往 官网 下载 go1.19.4.linux-amd64.tar.gz 1 2 3 tar -C /usr/local/ -xzf go1.19.4.linux-amd64.tar.gz export PATH=$PATH:/usr/local/go/bin go version 看到版本号代表 go 安装成功 编译器命令 1 2 3 4 5 6 7 8 9 10 11 12
2019-12-30
Redis
启动 Redis 1 2 3 4 redis-server /path/redis.conf # 指定配置文件启动 redis redis-cli # 开启 redis 客户端 systemctl restart redis.service # 重启 redis systemctl status redis # 检查 redis 运行状态 字符串 1 2
2019-12-24
C#
数据类型 类型 大小 举例 String 2 bytes/char s = “reference” bool 1 byte b = true char 2 bytes ch = ‘a’ byte 1 byte b = 0x78 short 2 bytes val = 70 int 4 bytes val = 700 long 8 bytes val
2019-7-13
Google 镜像站
通过 Nginx 反向代理搭建 Google 镜像站 Nginx 配置文件添加如下配置 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
2019-2-16
Core Dump
Core Dump 设置 生成 core 默认是不会产生 core 文件的 1 ulimit -c unlimited # -c 指定 core 文件的大小,unlimited 表示不限制 core 文件
2017-12-21
GDB
帮助信息 1 2 3 4 5 6 7 help # 列出命令分类 help running # 查看某个类别的帮助信息 help run # 查看命令 run 的帮助 help info # 列出查
2017-12-10
Batch
什么是批处理 批处理(Batch),也称为批处理脚本,批处理就是对某对象进行批量的处理 批处理文件的扩展
2017-11-5
Bash
常用快捷键 默认使用 Emacs 键位 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 CTRL+A # 移动到行
2017-4-21
赞赏
Wechat
Alipay
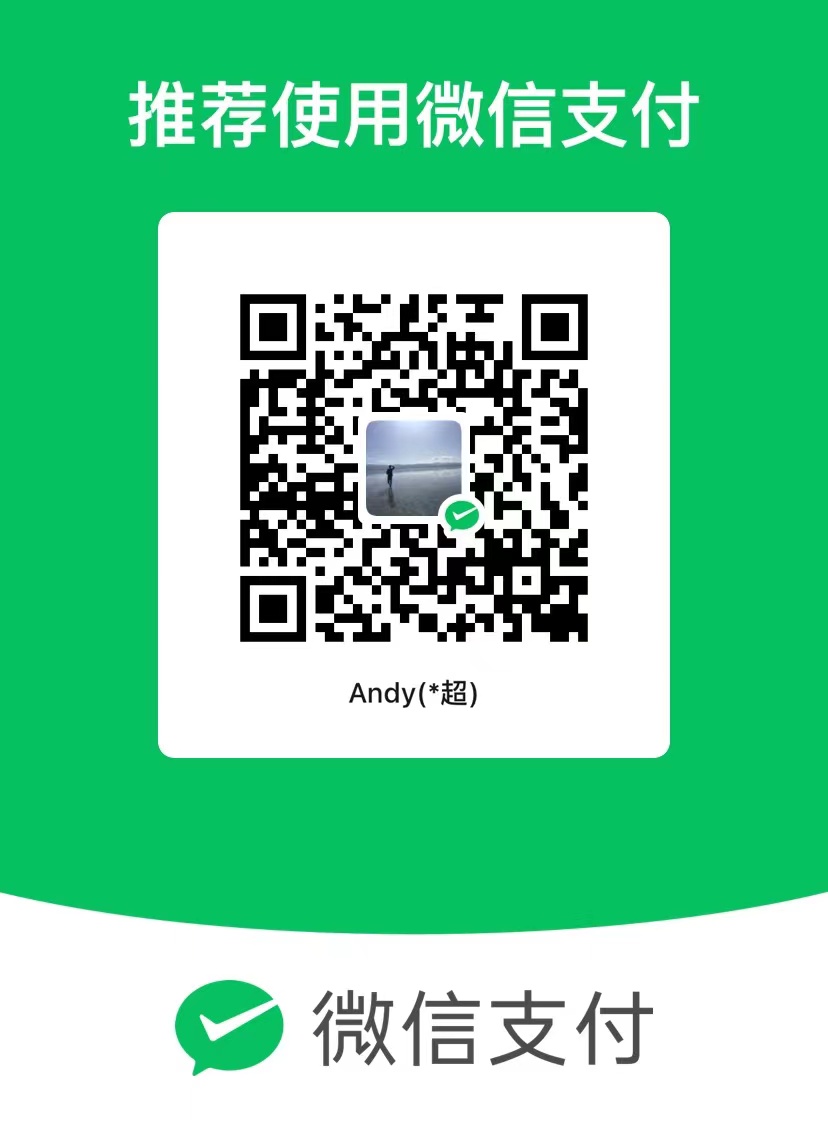